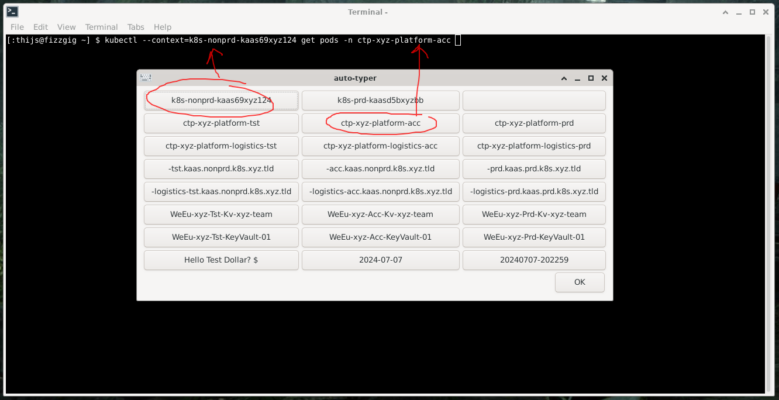
Auto-Typer – Linux Bash Script
TL;DR; A super simple Linux shell script to type a bunch of preset long “words” (only tested on Linux Mint XFCE).
At the office we work with Kubernetes clusters, and we are currently moving all of our stuff to a new cluster. The new cluster however has much longer namespace names, and longer domain names. This is quite tedious to have to type in, when using for example kubectl command line tools.
So I thought why not create a set of pre-cooked “words” to type, which I can select from a menu when hitting a special macro key…
So here it is. See this screenshot of my terminal, with the popup opened on top of it. Where I used the two circled buttons to help me type my command;
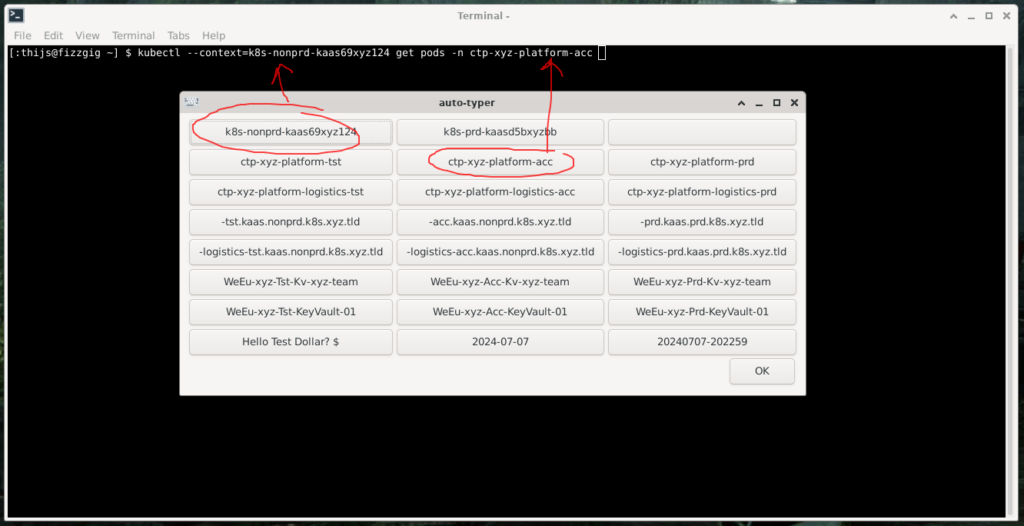
And here is the little shell script which makes it all work (I did anonymize the keyword set a bit, to remove work related references by xyz’s – you never know what people would be able to do with that):
#!/bin/bash
# Script to show a list of keywords to automatically type for you when clicked.
# Assign this script to any spare key you have to trigger the selection popup,
# or assign to a macro keyboard key.
#
# Dependencies: yad, xdotool, wmctrl, pkill, printf.
# Note: Only tested on Linux Mint with XFCE desktop.
# The choices to show, 3 values per row (if less, an empty button is shown).
# You can use dynamic shell expansion, like $(date), and placeholder {SPACE} will be replaced by a real space.
DATA=$(
cat <<SHERE
k8s-nonprd-kaas69xyz124 k8s-prd-kaasd5bxyzbb
ctp-xyz-platform-tst ctp-xyz-platform-acc ctp-xyz-platform-prd
ctp-xyz-platform-logistics-tst ctp-xyz-platform-logistics-acc ctp-xyz-platform-logistics-prd
-tst.kaas.nonprd.k8s.xyz.tld -acc.kaas.nonprd.k8s.xyz.tld -prd.kaas.prd.k8s.xyz.tld
-logistics-tst.kaas.nonprd.k8s.xyz.tld -logistics-acc.kaas.nonprd.k8s.xyz.tld -logistics-prd.kaas.prd.k8s.xyz.tld
WeEu-xyz-Tst-Kv-xyz-team WeEu-xyz-Acc-Kv-xyz-team WeEu-xyz-Prd-Kv-xyz-team
WeEu-xyz-Tst-KeyVault-01 WeEu-xyz-Acc-KeyVault-01 WeEu-xyz-Prd-KeyVault-01
Hello{SPACE}Test{SPACE}Dollar?{SPACE}\$ $(date +%Y-%m-%d) $(date +%Y%m%d-%H%M%S)
SHERE
)
# Remember which window to send the keystrokes to
export ACTIVE_WINDOW=$(printf "0x%0x" $(xdotool getactivewindow))
# Create a script file /tmp/do-type-MYPID which does screen focus changes, and keyboard typing.
TMP_SCRIPT="/tmp/do-type-$$"
cat << 'SHERE' > "${TMP_SCRIPT}"
#!/bin/bash
# Activate window to send keys to, type text, and close selection form.
wmctrl -a ${ACTIVE_WINDOW} -i
sleep 0.1
xdotool type -- "$*"
pkill -f auto-typer
SHERE
chmod 700 "${TMP_SCRIPT}"
# Split the data into 3 columns.
declare -a COL1 COL2 COL3
while read -r a b c; do
COL1+=("$a")
COL2+=("$b")
COL3+=("$c")
done <<< "$DATA"
# Convert the values to "--field" parameters for the "yad" tool.
FIELDS=()
for FIELD in "${COL1[@]}" "${COL2[@]}" "${COL3[@]}"; do
# If a value contains the "{SPACE}" keyword, replace it with a real space.
FIELD="${FIELD//\{SPACE\}/ }"
FIELDS+=("--field=${FIELD}:FBTN" "${TMP_SCRIPT} ${FIELD}")
done
# remove any older running instances
pkill -f auto-typer
# Construct the yad (selection form) command using an array.
yad_cmd=(
yad
--window-icon=org.xfce.settings.keyboard
--separator=" "
--borders=8
--title="auto-typer"
--columns=3
--form
--button="OK:0"
"${FIELDS[@]}"
)
# Execute the yad command to show the selection form.
"${yad_cmd[@]}"
# Remove tmp script
rm -rf "${TMP_SCRIPT}"
Code language: Bash (bash)
I have saved this script as ~/bin/words.sh, changed it’s mode to be executable: chmod 755 ~/bin/words.sh
, and assigned it to one of my macro-keys to trigger.
The script has lots of comments to explain what happens in case you want to find out what makes it tick.
The “DATA” set is a 3 column set of data, space separated. If you need a space in one of the values, then put placeholder {SPACE}
in there. As the data list is defined in a SHERE
document, you can use shell expansion in there, like the examples in the bottom line which render the current date as YYYY-MM-DD, or the date/time as YYYYMMDD-HHMMSS. This can be quite useful as well, to use in filenames.
And even if no-one will make use of the above script, it might give you some pointers in case you want to make something similar 😉
My next addition might be to add TOTP code auto-typing in there. To do that, I would need to be able to define a label and command as two separate items in the configuration. Not a big change, but I thought it would be better to keep the above code as simple as possible for now.
Thijs, July 7, 2024.
July 30, 2024; update:
To add the TOTP code option, I did replace this loop:
# Convert the values to "--field" parameters for the "yad" tool.
FIELDS=()
for FIELD in "${COL1[@]}" "${COL2[@]}" "${COL3[@]}"; do
# If a value contains the "{SPACE}" keyword, replace it with a real space.
FIELD="${FIELD//\{SPACE\}/ }"
FIELDS+=("--field=${FIELD}:FBTN" "${TMP_SCRIPT} ${FIELD}")
done
Code language: PHP (php)
By this:
# Convert the values to "--field" parameters for the "yad" tool.
FIELDS=()
for FIELD in "${COL1[@]}" "${COL2[@]}" "${COL3[@]}"; do
# If a value contains the "{SPACE}" keyword, replace it with a real space.
FIELD="${FIELD//\{SPACE\}/ }"
LABEL="${FIELD%###*}"
VALUE="${FIELD#*###}"
if [ "${LABEL}" != "${VALUE}" ]
then
LABEL="[${LABEL}]: ${VALUE}"
fi
FIELDS+=("--field=${LABEL}:FBTN" "${TMP_SCRIPT} ${VALUE}")
done
Code language: PHP (php)
And then in the “data” section at the top, add values like so:
MFA{SPACE}GITHUB###$( mintotp <<< HPSNFDTGS5PYB2WY ) MFA{SPACE}GANDI###$( mintotp <<< HPSNFDTGS5PYB2WY )
Code language: PHP (php)
You can add 3 in a row again if you want. The $( mintotp <<< HPSNFDTGS5PYB2WY )
will be expanded / executed into an actual TOTP code, using the $()
shell execution, and the mintotp
tool.
# for mintotp see: https://github.com/susam/mintotp
# install:
pip3 install mintotp
# example run:
mintotp <<< HPSNFDTGS5PYB2WY
700110
Code language: PHP (php)
The updated “for
” loop does split the value on the 3 hashes “###
” into two parts, a label at the left, and the value to type on the right. It then shows the value as [LABEL]: VALUE
, and on clicking just types the VALUE
. If there is no ###
in the data, it will act as previously, and regard the whole thing as VALUE
without showing a different label.
Warning/Note: the $()
expansion happens on opening the popup, for all lines at the same time. So they are NOT expanded when you press the button! You can use any $()
script, as long as it can be executed in advance to return some temporary semi-fixed value. For TOTP, you can use the code for around 60 seconds, so that’s fine. If you need something to execute a script only when pressing the button, then the code should be changed a bit.
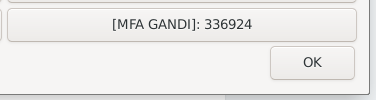
For completeness, here is a downloadable version of the new script, with TOTP option in it: